- ep.1 Player Movement
- ep.2 Scrolling ( Here Now )
- ep.3 Building
- ep.4 Enemy
- ep.5 Enemy Attack
- ep.6 Player Attack
Last time we got the player's movement all set, so now let's implement stage scrolling. As a warm-up, we'll create an off-screen handling process for when the player goes off-screen. Don't worry, we'll keep it super easy, so let's tackle this together!
Sprite 'Player'
First, open the 'Player' sprite.

Block Definition 'Off Screen'
Create a new block definition named 'Off Screen'.
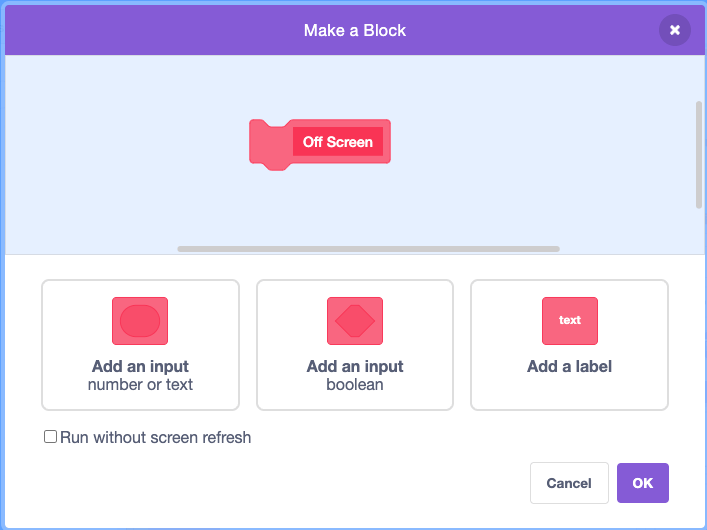
Place this block inside the forever loop under the green flag event.
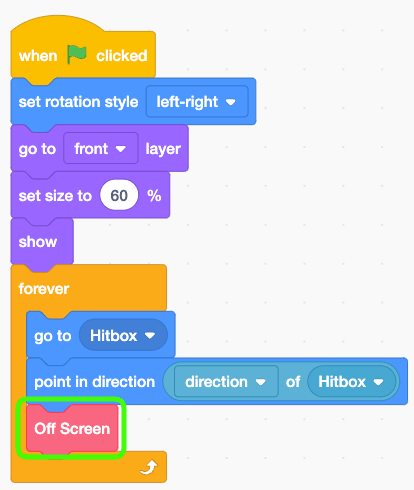
Place an 'if - else' block that includes an 'OR' condition.
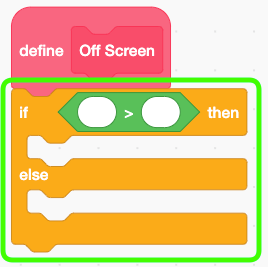
Let's check if the Y coordinate is greater than 180, meaning the player is jumping off the screen.
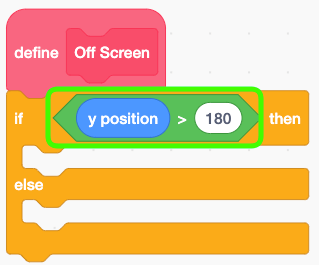
If true, set the ghost effect to 100, making the player invisible. If false, reset the ghost effect to 0. This way, the player appears to be jumping off the screen only while it's outside the visible area.
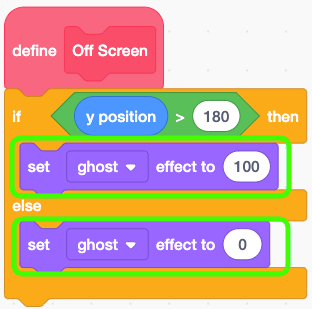
Preview
Awesome! Just this simple effect adds so much realism. It's great!
Stage
Next, let's create scrolling. Right now, the background stage doesn't move even when the player does, so it doesn't feel like you're moving through the world. By making the stage move to the left as the player moves to the right, we'll simulate scrolling and make the stage feel dynamic. Let's get that set up!
Open the 'Stage'.
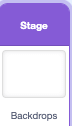
Variables: 'STAGE X' and 'STAGE SPEED X'
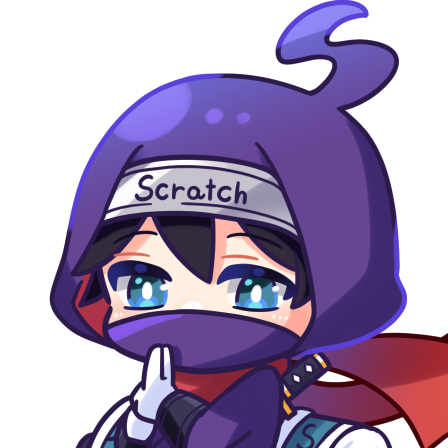
When you create a variable in the stage in Scratch, it automatically becomes a 'For all sprites' variable. It's a bit different since there are fewer checkboxes than usual, which might feel a little odd at first.
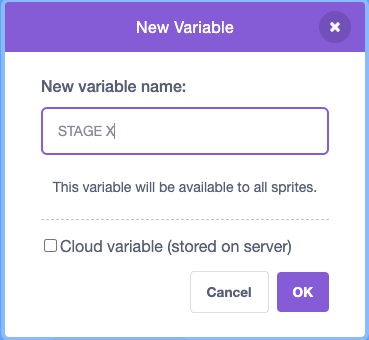
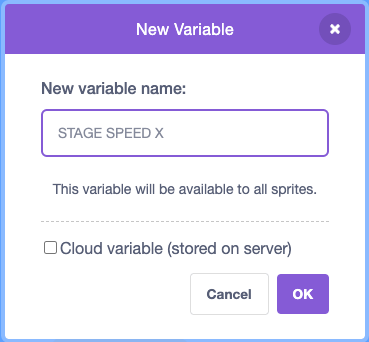
Initialize these variables like below.
- STAGE X = 0
- STAGE SPEED X = -2.5
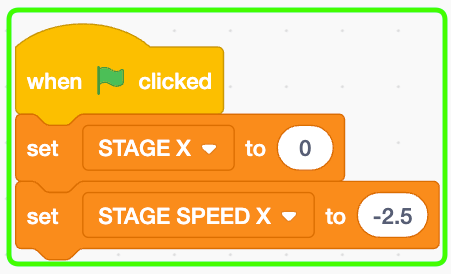
Place an forever block right below.
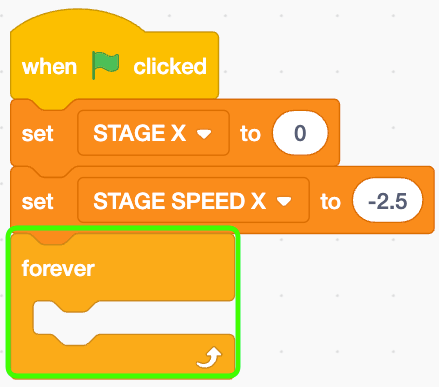
Messages: 'Prepare Scrolling' and 'Scroll'
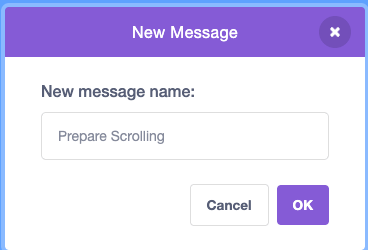
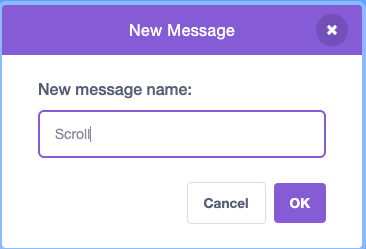
Place these blocks inside the loop.
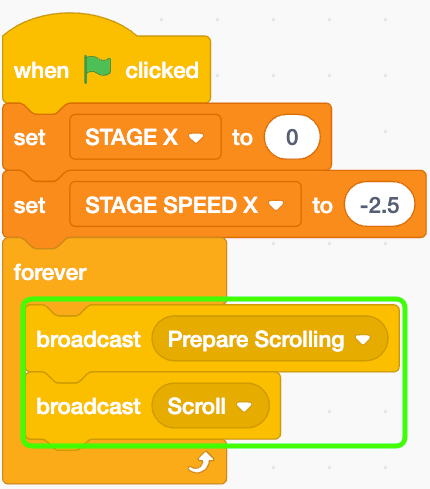
Alright, I'll come back later to add more implementations, but for now, the stage coding is all set.
Sprite 'Stage 1'
Now, open the sprite named "Stage 1." It might be a bit confusing because it shares a similar name with the background Stage, but this "Stage 1" is a sprite listed in your sprite library, not the background.

First, let's set it to show on the very back layer when you start.
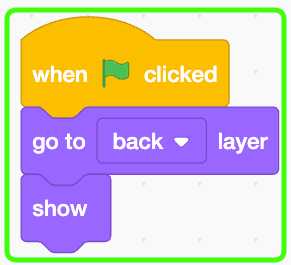
When I receive 'Preparing Scrolling', change 'STAGE X' by 'STAGE SPEED X'.
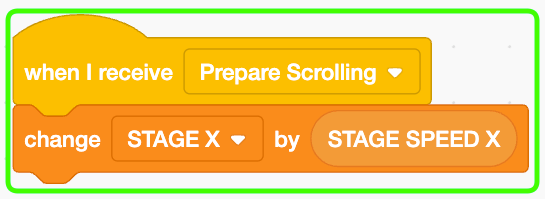
When I receive 'Scroll', set X to 'STAGE X' and Y to 0.
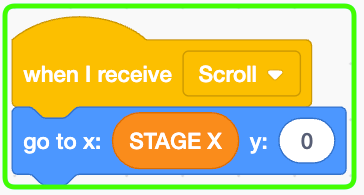
Place an 'if' block with the expression 'STAGE X = -480'.
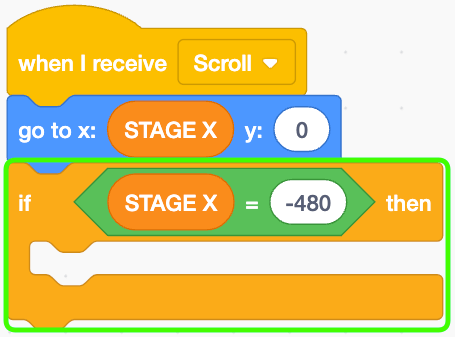
Change 'STAGE X' by 960 inside.
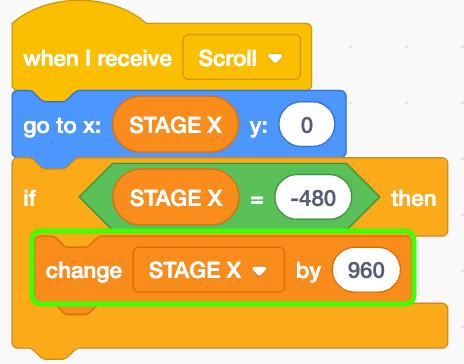
Alright, that's good for now. Refer to the screenshot below and drag and drop parts to duplicate them in the sprite "Stage 2."
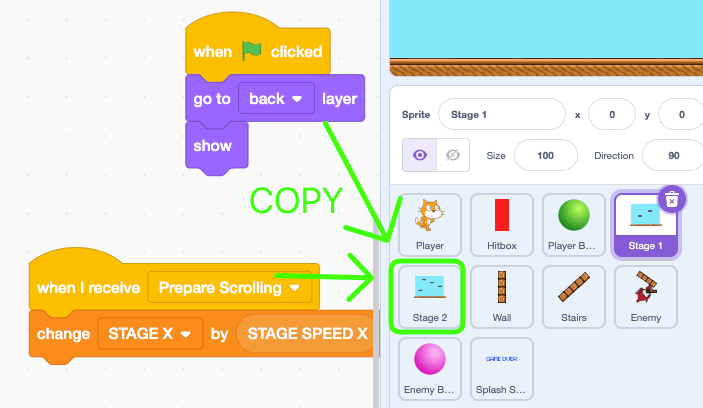
Sprite 'Stage 2'
Open the 'Stage 2' sprite.

Place an 'if-else' block that executes when it receives the 'Scroll' message.
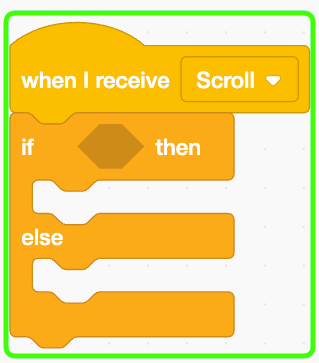
Check if 'STAGE X' is less than zero using a conditional statement.
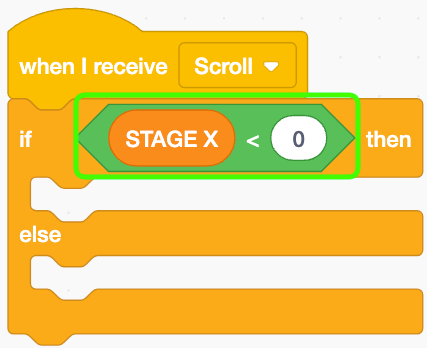
If true, perform addition to set X. If false, perform subtraction to set X.
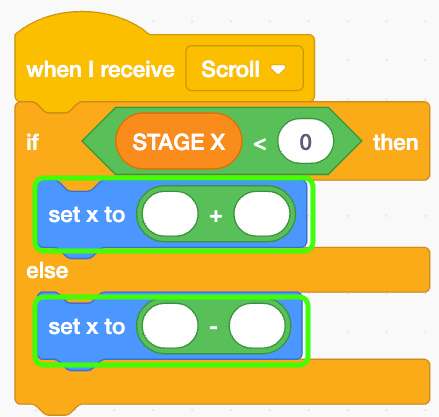
For addition, use the formula STAGE X + 480; for subtraction, use STAGE X - 480.
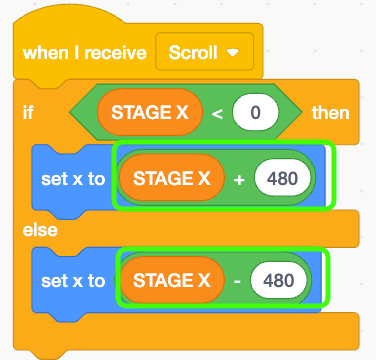
Preview
Wow, the stage has started to scroll! Even though the player isn't moving, the stage is scrolling, making it look like Scratch Cat is sliding on ice. While this is interesting, since we want the scrolling to be linked to the player's movement, let's add more to the implementation to achieve that.
Sprite 'Hitbox'
In this game, when the player's x position reaches -50, we'll stop moving the player on the screen and instead scroll the background.
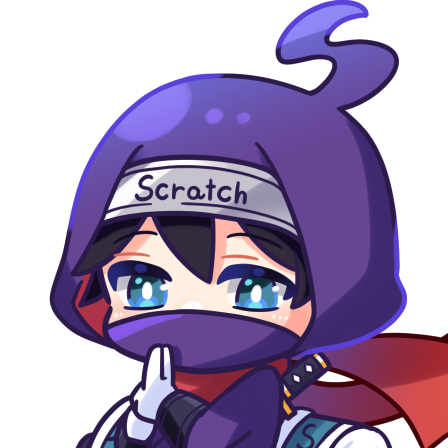
That means if the x position is between -240 and -50, the player can move, but the background doesn't scroll. Once it goes past -50, we'll keep the player's position fixed and only move the background. It sounds complex when explained, but the implementation is straightforward. Let's give it a shot!
Open the 'Hitbox' sprite.

Block Definition 'Scroll'
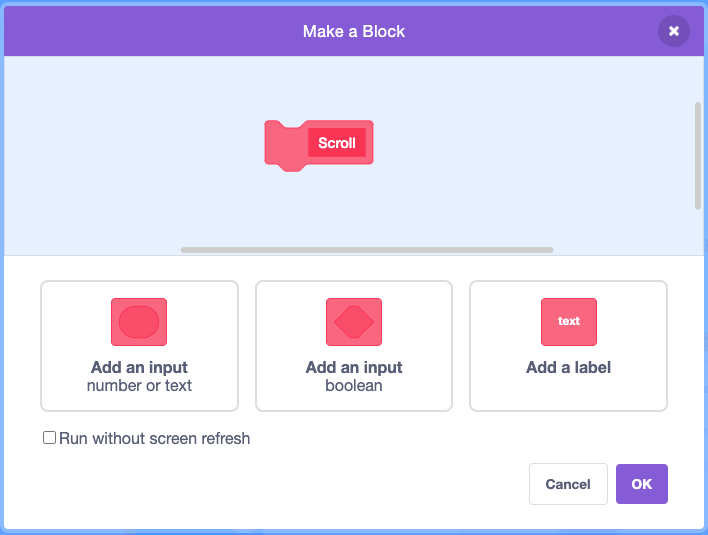
Place this block in the loop under the green flag.
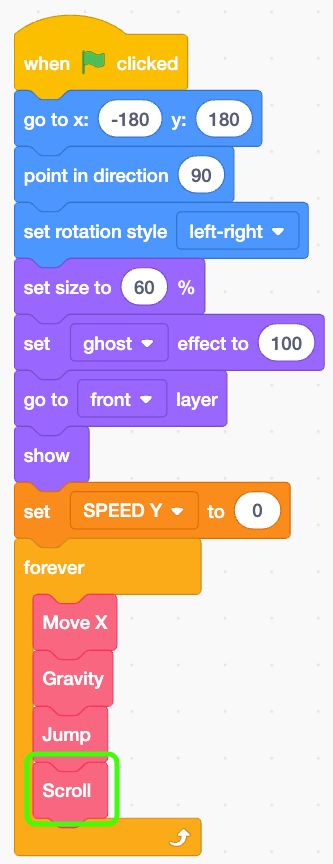
Let's check if the x position is greater than -50.
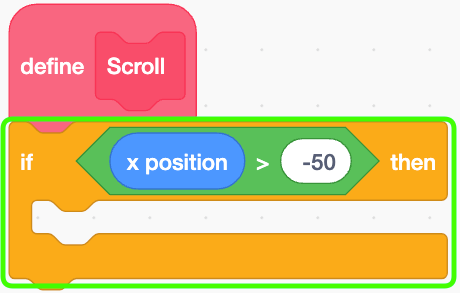
In this case, let's fix the x position at -50.
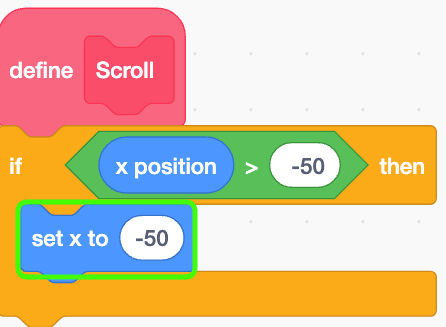
Stage
Open the Stage as the backdrop, not as a sprite.
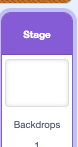
Surround the 'Preparing Scrolling' message with an 'if' block.
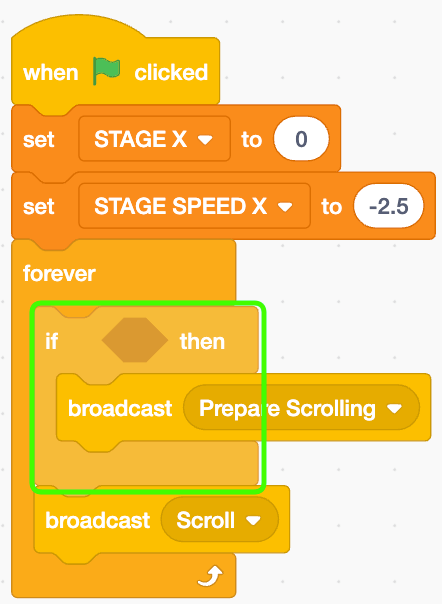
Let's check if the right arrow key is pressed.
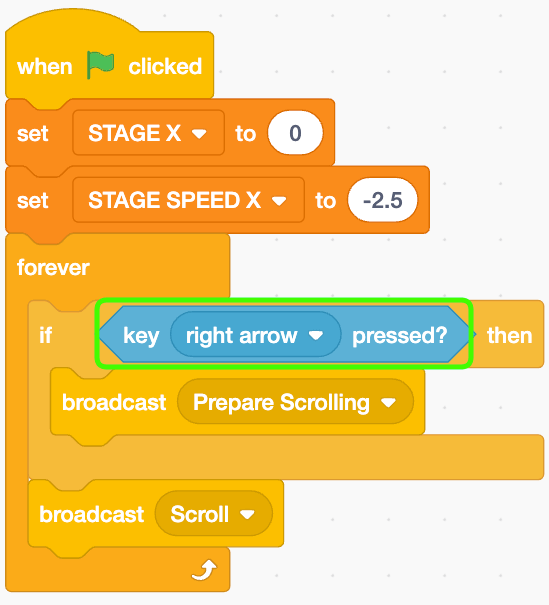
Let's add another 'if' block inside the first one. It's usually best not to nest too deeply, but up to three levels is fairly common.
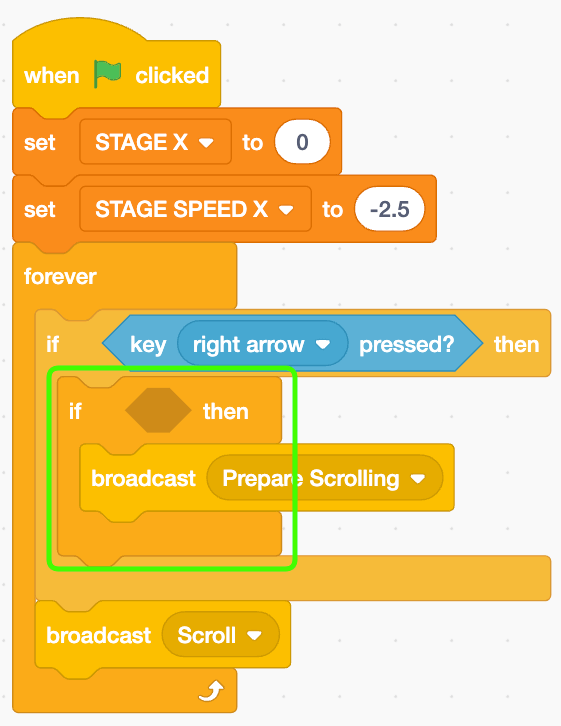
In this condition, check if the X position of the Hitbox sprite is -50.
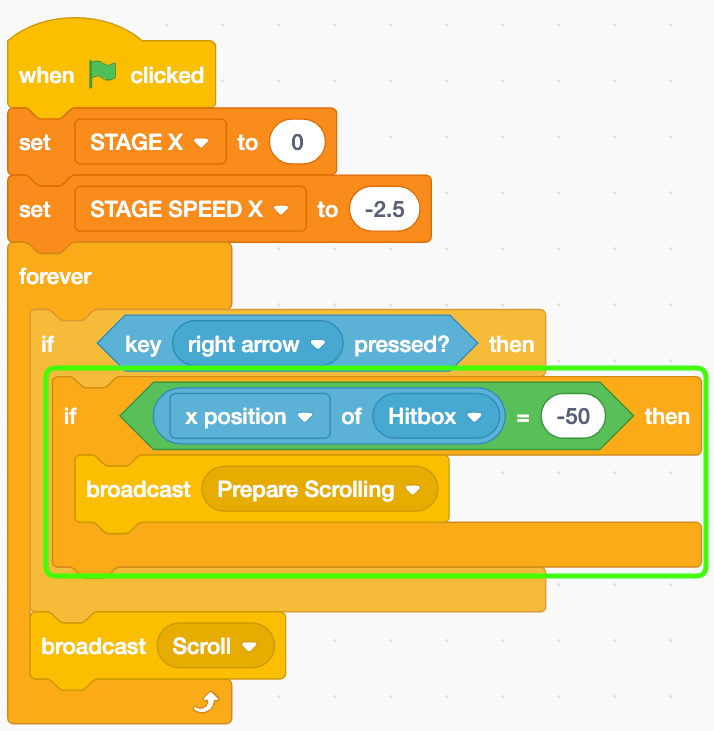
These two if blocks together will ensure that scrolling happens only when the right arrow key is pressed and the player is at the X position of -50. This setup fulfills the specification that the stage should scroll only under these conditions.
Preview
Hooray! The player's movements and the background scrolling are now linked together—what a great success! Now that the foundation of the game is set, let's move on to the highlight of Fortnite: implementing building mechanics. I'm excited to see where this goes!
- ep.1 Player Movement
- ep.2 Scrolling ( Here Now )
- ep.3 Building
- ep.4 Enemy
- ep.5 Enemy Attack
- ep.6 Player Attack
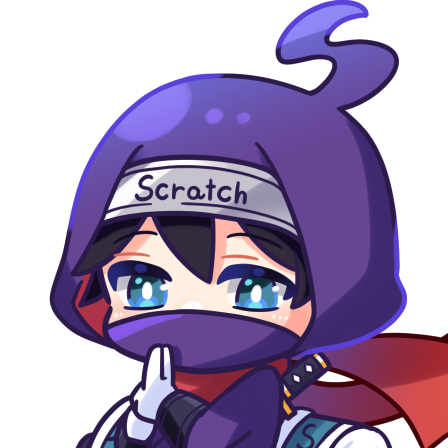
質問テンプレート(素早く3回クリックすると全選択できるのでコピーしよう)
・◯◯ ... 記事のどこまで実装が終わったのかを記入しよう。・□□ ... どんな問題が起きているのか、どういうときに起きるのか、具体的に書こう。
・共有済みURL ... たまに共有してない作品URLを書いてる人がいるけど、共有しないとこちらから確認できないからよろしくね。